p-on (or @)
p-on
is used to run python code when a specific event trigger.
You can use all events available in the DOM listed here : https://www.w3schools.com/tags/ref_eventattributes.asp
For example if you want to run an function on a click event just use p-on:click="..."
and put your function call inside.
<img :class="'rotate' if store.bob.rotate else ''" src="/images/bob.png"/><button p-on:click="store.bob.toggle_rotate()">Toggle Rotate</button>
from prune import Prune, notify
class Bob: def __init__(self): self.rotate = False
@notify def toggle_rotate(self): self.rotate = not self.rotate
Prune(bob=Bob())
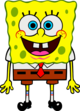
Shorthand syntax
Instead of writing p-on:click="..."
you can write @click="..."
.
Passing events as parameter
Sometimes you need data from an event, in this example we will change the scale of Bob thanks to a range input.
To send the input value to update_scale
we just have to write event.target.value
.
The event is accessible like in JS, it’s really the same thing.
from prune import Prune, notify
class Bob: def __init__(self): self.scale = 1
@notify def update_scale(self, new_scale:int): self.scale = new_scale
Prune(bob=Bob())
<img :style="f'transform: scale({store.bob.scale});'" src="/images/bob.png"/><input @input="store.bob.update_scale(event.target.value)" type="range" min="0" max="2" step="0.1" />
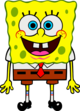